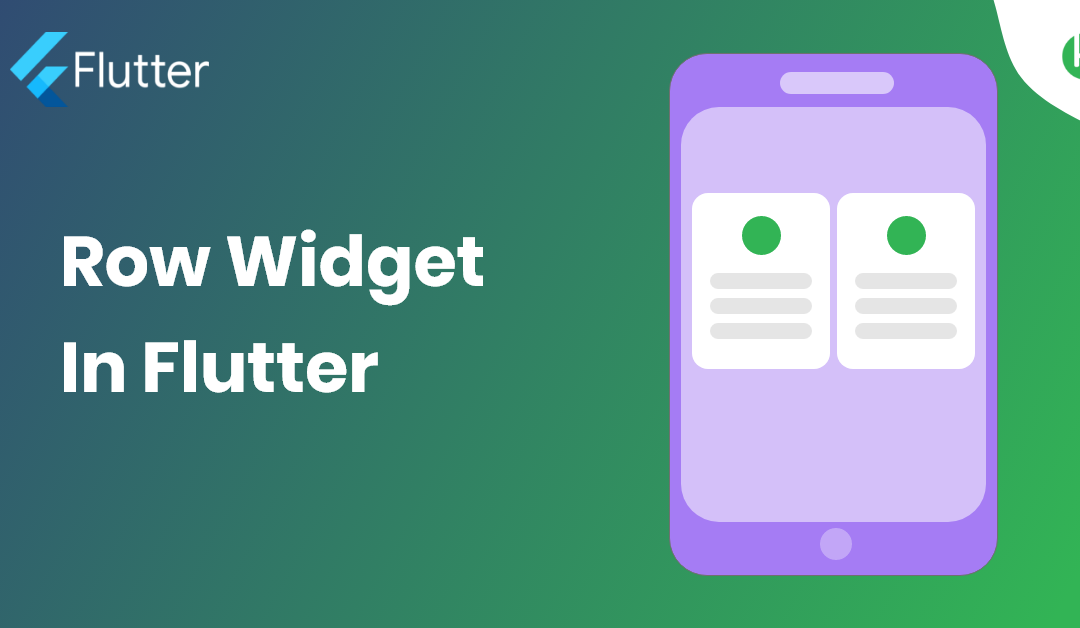
Row Widget In Flutter – A Short Guide
The Row widget in Flutter is a flexible layout widget that is used to arrange child widgets horizontally. It is one of the most basic and commonly used widgets in Flutter and is an essential tool for building responsive and attractive user interfaces. In this blog post, we will explore the basics of using the Row widget in Flutter and show you how to create and customize it for your app.
To create a Row widget in Flutter, simply wrap the child widgets you want to display in a Row widget. For example:
Row( children: [ Text('Row Item 1'), Text('Row Item 2'), Text('Row Item 3'), ], )
In the above example, we are creating a Row widget with three child Text widgets. The child widgets will be displayed horizontally, one after the other.
The Row widget provides several properties that you can use to customize its appearance and behavior. For example, you can use the mainAxisAlignment property to control the alignment of the child widgets in the main axis. For example:
Row( mainAxisAlignment: MainAxisAlignment.spaceBetween, children: [ Text('Row Item 1'), Text('Row Item 2'), Text('Row Item 3'), ], )
In the above example, we are using the mainAxisAlignment property to distribute the space evenly between the child widgets in the main axis.
You can also use the crossAxisAlignment property to control the alignment of the child widgets in the cross axis. For example:
Row( crossAxisAlignment: CrossAxisAlignment.center, children: [ Text('Row Item 1'), Text('Row Item 2'), Text('Row Item 3'), ], )
In the above example, we are using the crossAxisAlignment property to center the child widgets in the cross-axis.
In conclusion, the Row widget in Flutter is a simple and flexible layout widget that is essential for building responsive and attractive user interfaces. With the ability to customize its appearance and behavior, it makes it a great option for creating engaging and interactive experiences in your app.
You can have in detail information on the Row widget in this official Flutter Channel.